39. Summary
You will need to practice! Use this FREE site to practice the skills you see in this course.
Below is a review of the things you learned in this lesson. I know when I was getting started, I always wanted more practice. This course will move pretty fast from this point forward, so if you feel like you just want more practice problems on a topic, there are a number of free courses on the web. Two that you should definitely take advantage of (for the benefit of this program, but also for finding a job afterward) are HackerRank and Codewars. Within each of these, you should create a profile and work on mastering Python! I was obsessed with these websites for getting all the practice I needed when I first started programming. As you get better, you can advance to harder problems and sites with even greater challenges. Happy coding!
Lesson Summary
You learned a ton in this lesson - here are a few of the big ideas to make sure you take them with you!
Data Structures
There are a number of built in python data structures that you will use all the time when programming. You can find a table of them available below:
Data Structure | Ordered | Mutable | Constructor | Example |
---|---|---|---|---|
int | NA | NA | int() |
5 |
float | NA | NA | float() |
6.5 |
string | Yes | No | ' ' or " " or str() |
"this is a string" |
bool | NA | NA | NA | True or False |
list | Yes | Yes | [ ] or list() |
[5, 'yes', 5.7] |
tuple | Yes | No | ( ) or tuple() |
(5, 'yes', 5.7) |
set | No | Yes | { } or set() |
{5, 'yes', 5.7} |
dictionary | No | Keys: No | { } or dict() |
{'Jun':75, 'Jul':89} |
Mathematical, Comparison, and Logical Operators
You also learned about mathematical operators, as shown in the table below and logical operators shown in the table below that! Awesome job!
Mathematical Operators
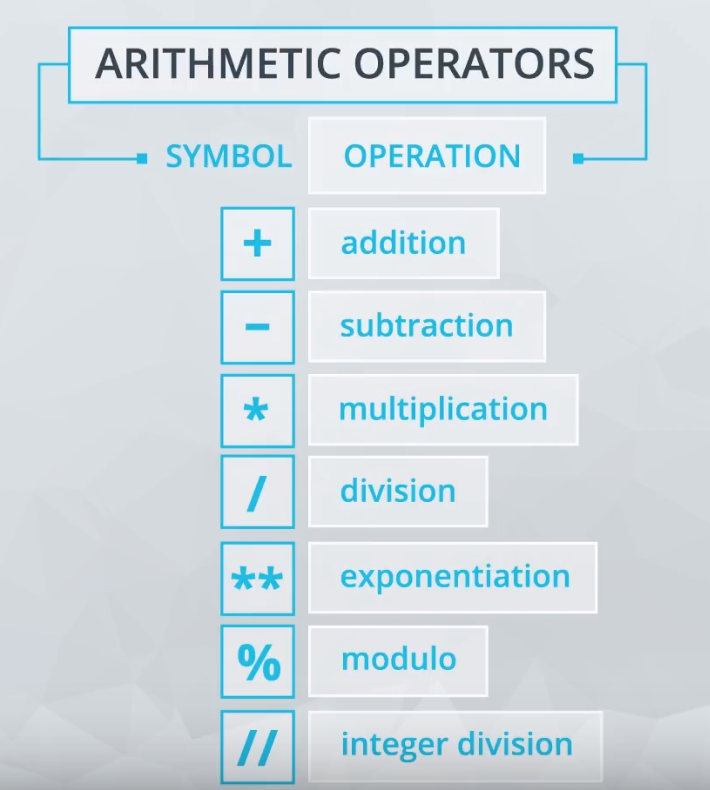
Comparison and Logical Operators
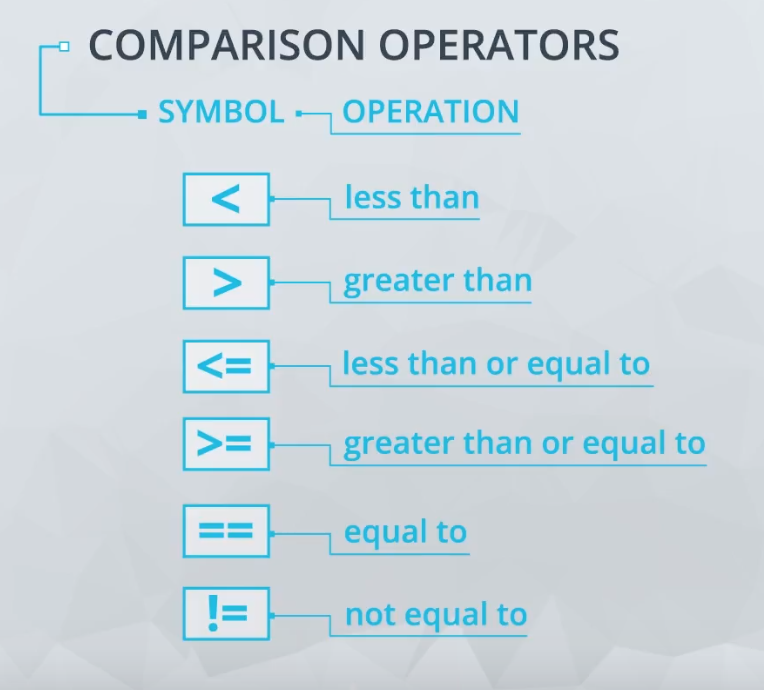
Booleans, Comparison Operators, and Logical Operators
The bool data type holds one of the values True
or False
, which are often encoded as 1
or 0
, respectively.
There are 6 comparison operators that are common to see in order to obtain a bool
value:
Comparison Operators
Symbol Use Case | Bool | Operation |
---|---|---|
5 < 3 | False | Less Than |
5 > 3 | True | Greater Than |
3 <= 3 | True | Less Than or Equal To |
3 >= 5 | False | Greater Than or Equal To |
3 == 5 | False | Equal To |
3 != 5 | True | Not Equal To |
And there are three logical operators you need to be familiar with:
Logical Use | Bool | Operation |
---|---|---|
5 < 3 and 5 == 5 |
False | and - Evaluates if all provided statements are True |
5 < 3 or 5 == 5 |
True | or - Evaluates if at least one of many statements is True |
not 5 < 3 |
True | not - Flips the Bool Value |
Here is more information on how George Boole changed the world!
At this point, you have learned a lot of skills to build on. The next lessons move pretty quickly to help you understand a number of coding practices that you will see in a job, as well as help you get prepared for the project.